- Sublime Text C++ Compiler Machine
- Sublime Text C++ Compiler Mac
- Sublime Text C++ Compiler Macro
- Sublime Text C
New instructions: https://chromium.googlesource.com/chromium/src/+/master/docs/sublime_ide.mdContents
- 9 Example plugin
Contents
- 9 Example plugin
Sublime Text is much lighter than Xcode because ST is not a full featured IDE, likc Xcode. What you'll end up doing is installing/using gcc or g directly via the command line (which happens to be my preferred way of working). Command line compiling can get to be tedious though with all of the parameters that need to be passed. I am learning C at college now, and teachers told me to use codeblocks as an IDE, but in my opinion codeblocks is a bit ugly and that's why I've chosen Sublime Text 2, the BEST IDE/Text Editor out there. At the moment I write my code via sublime, save it and then compile it via mac os terminal (gcc) and than run it on the terminal as well. Sublime Text 4 is the current version of Sublime Text. For bleeding-edge releases, see the dev builds. Sublime Text may be downloaded and evaluated for free, however a license must be purchased for continued use. There is currently no enforced time limit for the evaluation. /. Online C Compiler. Code, Compile, Run and Debug C program online. Write your code in this editor and press 'Run' button to compile and execute it.
What is Sublime Text?
- Project support.
- Theme support.
- Works on Mac, Windows and Linux.
- No need to close and re-open during a
gclient sync
. - Supports many of the great editing features found in popular IDE's like Visual Studio, Eclipse and SlickEdit.
- Doesn't go to lunch while you're typing.
- The UI and keyboard shortcuts are pretty standard (e.g. saving a file is still Ctrl+S on Windows).
- It's inexpensive and you can evaluate it (fully functional) for free.
Installing Sublime Text 2
Preferences
'tab_size': 2,
Project files
'folders':
{
}
}
'folders':
{
'name': 'src',
'*.vcproj',
'*.sln',
'*.gitmodules',
],
'build',
'third_party',
'Debug',
]
]
Navigating the project
- 'Goto Anything' or Ctrl+P is how you can quickly open a file or go to a definition of a type such as a class. Just press Ctrl+P and start typing.
- Open source/header file: If you're in a header file, press Alt+O to open up the corresponding source file and vice versa. For more similar features check out the Goto->Switch File submenu.
- 'Go to definition': Right click a symbol and select 'Navigate to Definition'. A more powerful way to navigate symbols is by using the Ctags extension and use the Ctrl+T,Ctrl+T shortcut. See the section about source code indexing below.
Enable source code indexing
- Install the Sublime Package Control package: https://packagecontrol.io/installation
- Install Exuberant Ctags and make sure that ctags is in your path: http://ctags.sourceforge.net/
- On linux you should be able to just do: sudo apt-get install ctags
- Install the Ctags plugin: Ctrl+Shift+P and type 'Package Control: Install Package'
- Create a batch file (e.g. ctags_builder.bat) that you can run either manually or automatically after you do a gclient sync:This takes a couple of minutes to run, but you can work while it is indexing.
ctags --languages=C++ --exclude=third_party --exclude=.git --exclude=build --exclude=out -R -f .tmp_tags & ctags --languages=C++ -a -R -f .tmp_tags third_partyplatformsdk_win8 & ctags --languages=C++ -a -R -f .tmp_tags third_partyWebKit & move /Y .tmp_tags .tags
- Edit the CTags.sublime-settings file for the ctags plugin so that it runs ctags with the above parameters. Note: the above is a batch file - don't simply copy all of it verbatim and paste it into the CTags settings file :-)
Windows: git config --global core.excludesfile %USERPROFILE%.gitignore
Mac, Linux: git config --global core.excludesfile ~/.gitignoreBuilding with ninja
'cmd': ['ninja', '-C', 'outDebug', 'chrome.exe'],
'file_regex': '^[./]*([a-z]?:?[w./]+)[(:]([0-9]+)[):,]([0-9]+)?[:)]?(.*)$'
You can also add build variants so that you can also have quick access to building other targets like unit_tests or browser_tests. You build description file could look like this:
And keep using 'ctrl+b' for a regular, 'chrome.exe' build. Enjoy!
Example plugin
import subprocess
class RunLintCommand(sublime_plugin.TextCommand):
command = ['cpplint.bat', self.view.file_name()]
Sublime Text C++ Compiler Machine
stdout=subprocess.PIPE,
print process.communicate()[1]
{
}
D:srccgitsrccontentbrowserbrowsing_instance.cc:69: Add #include <string> for string [build/include_what_you_use] [4]
Done processing D:srccgitsrccontentbrowserbrowsing_instance.cc
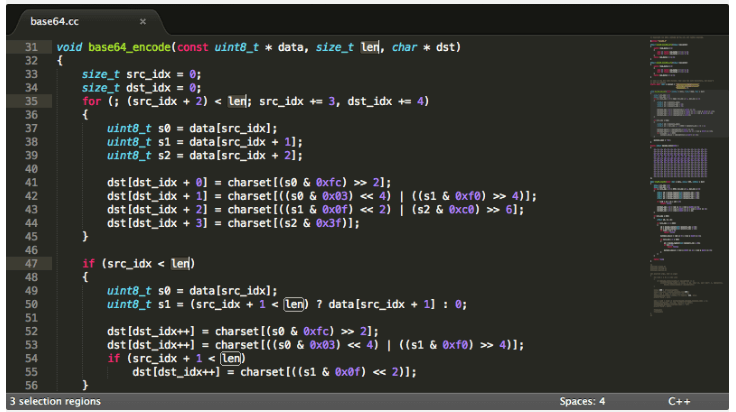
['__class__', '__delattr__', '__dict__', '__doc__', '__format__', '__getattribute__', '__hash__', '__init__', '__len__', '__module__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'add_regions', 'begin_edit', 'buffer_id', 'classify', 'command_history', 'em_width', 'encoding', 'end_edit', 'erase', 'erase_regions', 'erase_status', 'extract_completions', 'extract_scope', ... <snip>
Compile current file using Ninja
As a more complex plug in example, look at the attached python file: compile_current_file.py. This plugin will compile the current file with Ninja, so will start by making sure that all this file's project depends on has been built before, and then build only that file.
First, it confirms that the file is indeed part of the current project (by making sure it's under the <project_root> folder, which is taken from the self.view.window().folders() array, the first one seems to always be the project folder when one is loaded). Then it looks for the file in all the .ninja build files under the <project_root>out<target_build>, where <target_build> must be specified as an argument to the compile_current_file command. Using the proper target for this file compilation, it starts Ninja from a background thread and send the results to the output.exec panel (the same one used by the build system of Sublime Text 2). So you can use key bindings like these two, to build the current file in either Debug or Release mode:
{ 'keys': ['ctrl+f7'], 'command': 'compile_current_file', 'args': {'target_build': 'Debug'} },
{ 'keys': ['ctrl+shift+f7'], 'command': 'compile_current_file', 'args': {'target_build': 'Release'} },
If you are having trouble with this plugin, you can set the python logging level to DEBUG in the console and see some debug output.
Format selection (or area around cursor) using clang-format
Miscellaneous tips
- To synchronize the project sidebar with the currently open file, right click in the text editor and select 'Reveal in Side Bar'. Alternatively you can install the SyncedSideBar sublime package (via the Package Manager) to have this happen automatically like in Eclipse.
- If you're used to hitting a key combination to trigger a build (e.g. Ctrl+Shift+B in Visual Studio) and would like to continue to do so, add this to your Preferences->Key Bindings - User file:
- { 'keys': ['ctrl+shift+b'], 'command': 'show_panel', 'args': {'panel': 'output.exec'} }
- Install the Open-Include plugin (Ctrl+Shift+P, type:'Install Package', type:'Open Include'). Then just put your cursor inside an #include path, hit Alt+D and voila, you're there.
- If you want to take that a step further, add an entry to the right-click context menu by creating a text file named 'context.sublime-menu' under '%APPDATA%Sublime Text 2PackagesUser' with the following content:
[ { 'command': 'open_include', 'caption': 'Open Include' } ]
- Open Command Palette (Ctrl-Shift-P)
- Type 'Package Control: Install Package' (note: given ST's string match is amazing you can just type something like 'instp' and it will find it :-)).
- Case Conversion (automatically swap casing of selected text -- works marvel with multi-select -- go from a kConstantNames to ENUM_NAMES in seconds)
- CTags (see detailed setup info above).
- Git
- Open-Include
- Text Pastry (insert incremental number sequences with multi-select, etc.)
- Wrap Plus (auto-wrap a comment block to 80 columns with Alt-Q)
The text editor called Sublime Text, the compiler Clang, and your Mac terminal can all work together so you can write simple programs on your Mac. I am running on the assumption for now that you have already installed Clang and Sublime Text on your computer.
Use the Power of Three for Writing & Compiling in C:
Write a Program in Sublime Text
Go ahead and write something in C, perhaps a “Hello World,” as this is always a good start.
Save your Sublime Text file to your location of choice in your computer. For example, I saved my file as ‘helloworld.c’ and saved it to a folder called ‘workspace_cpp’.
Sublime Text C++ Compiler Mac
Use Your Terminal
In your terminal, navigate to the folder your file is in. Type ‘clang’ and the file name, in this case, ‘helloworld.c’
Nothing occurs yet, since clang was simply used to compile the program.
Alternatively, the shortcut is to type in the command: ‘make’ and the file name (without the file type).
To run the program, type into your terminal ‘.a/.out’
The output will print on the terminal. In my case, the next line on my terminal looks like this:

Sublime Text C++ Compiler Macro
Everytime you make changes to your program, remember, you will need to re-compile your program with clang, and then re-run your program.
Sublime Text C
The Computer Science of it All
Source code –> compiler –> machine code
Source code is the input, such as the Hello World program. The compiler is the algorithm, in the form of software. The output is the machine code, or binary (0’s and 1’s).
Clang, as noted above, is a compiler. It’s short for “C Language.”
.a/.out runs the program, and is specifically a file format. It is short for “assembler output.”